COUNTER - Up/Down pulse counter
Each counter block, when enabled, can count up/down with user-defined step value on the rising edge on input trigger. The counters can also be initialised to a user-defined START value.
Fields
Name |
Type |
Description |
---|---|---|
ENABLE |
bit_mux |
Halt on falling edge, reset and enable on rising |
TRIG |
bit_mux |
Rising edge ticks the counter up/down by STEP |
DIR |
bit_mux |
Up/Down direction (0 = Up, 1 = Down) |
TRIG_EDGE |
param enum |
INP trigger edge
0 Rising
1 Falling
2 Either
|
OUT_MODE |
param enum |
Counter OUT update mode (on internal counter value change or on ENABLE falling edge)
0 On-Change
1 On-Disable
|
SET |
param int |
set current value of a counter |
START |
param int |
Counter start value |
STEP |
param |
Up/Down step value |
MAX |
param int |
Rollover value |
MIN |
param int |
Value to which counter should rollover to |
CARRY |
bit_out |
Internal counter overflow status |
OUT |
pos_out |
Current counter value |
Counting pulses
The most common use of a counter block is when you would like to track the number of trigger edges received while enabled:
(Source code, png, hires.png, pdf)
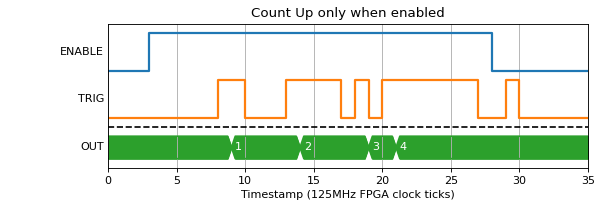
The TRIG_EDGE field can be used to select the track on rising, falling, or both edges:
(Source code, png, hires.png, pdf)
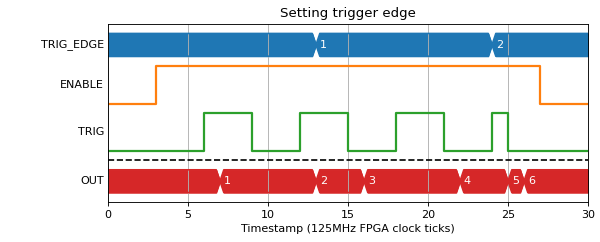
You can also set the start value to be loaded on enable, and step up by a number other than one:
(Source code, png, hires.png, pdf)
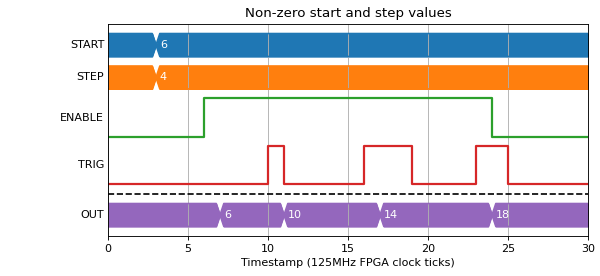
You can also set the direction that a pulse should apply step, so it becomes an up/down counter. The direction is sampled on the same clock tick as the pulse edge:
(Source code, png, hires.png, pdf)
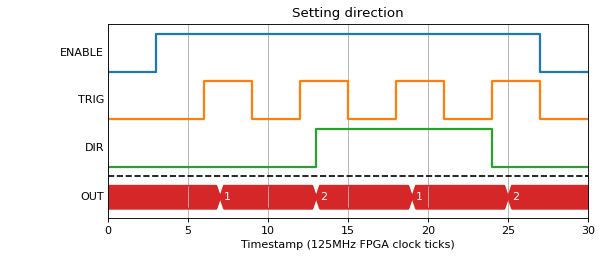
When the OUT_MODE is set to On-Disable, the OUT output will only be changed to the internal counter value on ENABLE’s falling edge:
(Source code, png, hires.png, pdf)
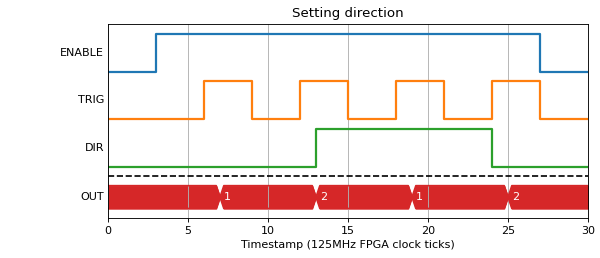
Rollover
If the count goes higher than the max value for an int32 (2147483647) the CARRY output gets set high and the counter rolls. The CARRY output stays high for as long as the trigger input stays high.
(Source code, png, hires.png, pdf)
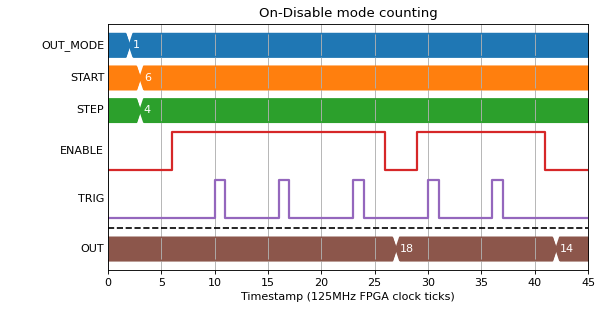
A similar thing happens for a negative overflow:
(Source code, png, hires.png, pdf)
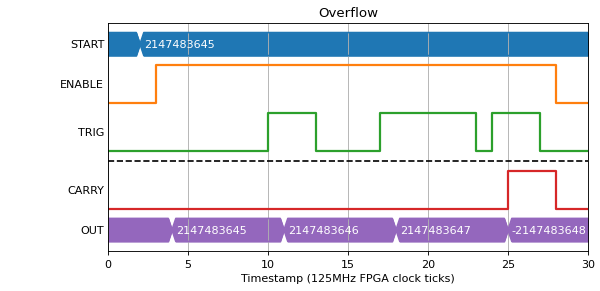
When the OUT_MODE is set to On-Disable, the CARRY output will get set to high on ENABLE’s falling edge if any overflow produced while the counter was enabled:
(Source code, png, hires.png, pdf)
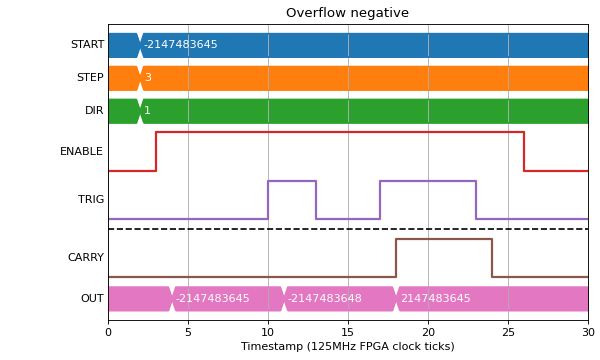
Edge cases
If the Enable input goes low at the same time as a trigger, there will be no output value on the next clock tick.
(Source code, png, hires.png, pdf)
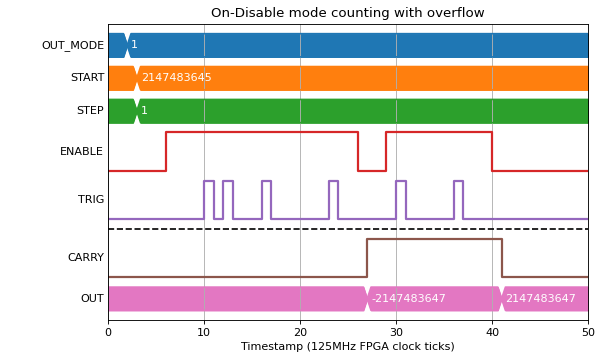
If the step size is changed at the same time as a trigger input edge, the output value for that trigger will be the new step size.
(Source code, png, hires.png, pdf)
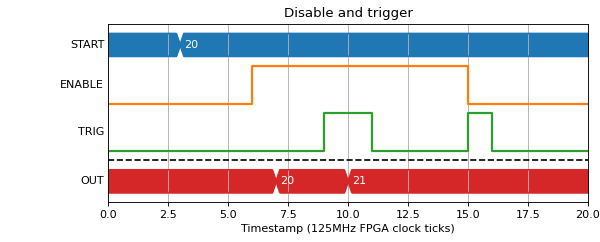