SEQ - Sequencer
The sequencer block performs automatic execution of sequenced lines to produce timing signals. Each line optionally waits for an external trigger condition and runs for an optional phase1, then a mandatory phase2 before moving to the next line. Each line sets the block outputs during phase1 and phase2 as defined by user-configured mask. Individual lines can be repeated, and the whole table can be repeated, with a value of 0 meaning repeat forever.
Fields
Name |
Type |
Description |
---|---|---|
ENABLE |
bit_mux |
Stop on falling edge, reset and enable on rising edge |
BITA |
bit_mux |
BITA for optional trigger condition |
BITB |
bit_mux |
BITB for optional trigger condition |
BITC |
bit_mux |
BITC for optional trigger condition |
POSA |
pos_mux |
POSA for optional trigger condition |
POSB |
pos_mux |
POSB for optional trigger condition |
POSC |
pos_mux |
POSC for optional trigger condition |
TABLE |
table short |
Sequencer table of lines
REPEATS Number of times the line will repeat
TRIGGER The trigger condition to start the phases
POSITION The position that can be used in trigger condition
TIME1 The time the optional phase 1 should take
OUTA1 Output A value during phase 1
OUTB1 Output B value during phase 1
OUTC1 Output C value during phase 1
OUTD1 Output D value during phase 1
OUTE1 Output E value during phase 1
OUTF1 Output F value during phase 1
TIME2 The time the mandatory phase 2 should take
OUTA2 Output A value during phase 2
OUTB2 Output B value during phase 2
OUTC2 Output C value during phase 2
OUTD2 Output D value during phase 2
OUTE2 Output E value during phase 2
OUTF2 Output F value during phase 2
15:0 REPEATS
19:16 TRIGGER enum
0 Immediate
1 BITA=0
2 BITA=1
3 BITB=0
4 BITB=1
5 BITC=0
6 BITC=1
7 POSA>=POSITION
8 POSA<=POSITION
9 POSB>=POSITION
10 POSB<=POSITION
11 POSC>=POSITION
12 POSC<=POSITION
63:32 POSITION int
95:64 TIME1
20:20 OUTA1
21:21 OUTB1
22:22 OUTC1
23:23 OUTD1
24:24 OUTE1
25:25 OUTF1
127:96 TIME2
26:26 OUTA2
27:27 OUTB2
28:28 OUTC2
29:29 OUTD2
30:30 OUTE2
31:31 OUTF2
|
PRESCALE |
param time |
Prescalar for sequencer table times |
REPEATS |
param |
Number of times the table will repeat |
ACTIVE |
bit_out |
Sequencer active flag |
OUTA |
bit_out |
Output A for phase outputs |
OUTB |
bit_out |
Output B for phase outputs |
OUTC |
bit_out |
Output C for phase outputs |
OUTD |
bit_out |
Output D for phase outputs |
OUTE |
bit_out |
Output E for phase outputs |
OUTF |
bit_out |
Output F for phase outputs |
TABLE_REPEAT |
read |
Current iteration through the entire table |
TABLE_LINE |
read |
Current line in the table that is active |
LINE_REPEAT |
read |
Current iteration of the active table line |
STATE |
read enum |
Internal statemachine state
0 UNREADY
1 WAIT_ENABLE
2 WAIT_TRIGGER
3 PHASE1
4 PHASE2
|
HEALTH |
read enum |
Was last run successful?
0 OK
1 Next table not written in time
2 Table overwritten while in used
|
CAN_WRITE_NEXT |
read uint 1 |
Next table can be written in continuous mode |
Sequencer Table Line Composition
Bit Field |
Name |
Description |
---|---|---|
[15:0] |
REPEATS |
Number of times the line will repeat |
[19:16] |
TRIGGER |
The trigger condition to start the phases
0: Immediate
1: BITA=0
2: BITA=1
3: BITB=0
4: BITB=1
5: BITC=0
6: BITC=1
7: POSA>=POSITION
8: POSA<=POSITION
9: POSB>=POSITION
10: POSB<=POSITION
11: POSC>=POSITION
12: POSC<=POSITION
|
[63:32] |
POSITION |
The position that can be used in trigger condition |
[95:64] |
TIME1 |
The time the optional phase 1 should take |
[20:20] |
OUTA1 |
Output A value during phase 1 |
[21:21] |
OUTB1 |
Output B value during phase 1 |
[22:22] |
OUTC1 |
Output C value during phase 1 |
[23:23] |
OUTD1 |
Output D value during phase 1 |
[24:24] |
OUTE1 |
Output E value during phase 1 |
[25:25] |
OUTF1 |
Output F value during phase 1 |
[127:96] |
TIME2 |
The time the mandatory phase 2 should take |
[26:26] |
OUTA2 |
Output A value during phase 2 |
[27:27] |
OUTB2 |
Output B value during phase 2 |
[28:28] |
OUTC2 |
Output C value during phase 2 |
[29:29] |
OUTD2 |
Output D value during phase 2 |
[30:30] |
OUTE2 |
Output E value during phase 2 |
[31:31] |
OUTF2 |
Output F value during phase 2 |
Generating fixed pulse trains
The basic use case is for generating fixed pulse trains when enabled. For example we can ask for 3x 50% duty cycle pulses by writing a single line table that is repeated 3 times. When enabled it will become active and immediately start producing pulses, remaining active until the pulses have been produced:
(Source code
, png
, hires.png
, pdf
)
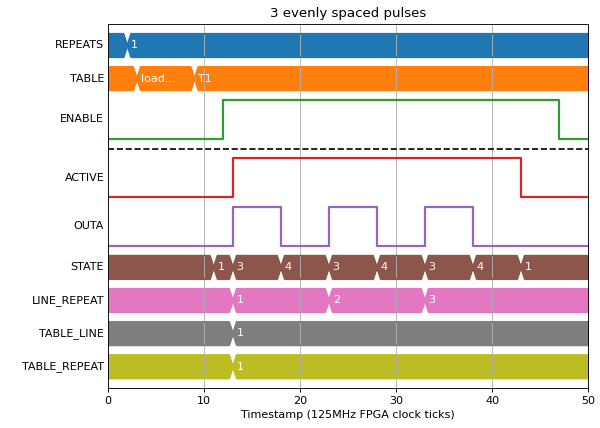
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
3 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
5 |
0 |
0 |
0 |
0 |
0 |
0 |
We can also use it to generate irregular streams of pulses on different outputs by adding more lines to the table. Note that OUTB which was high at the end of Phase2 of the first line remains high in Phase1 of the second line:
(Source code
, png
, hires.png
, pdf
)
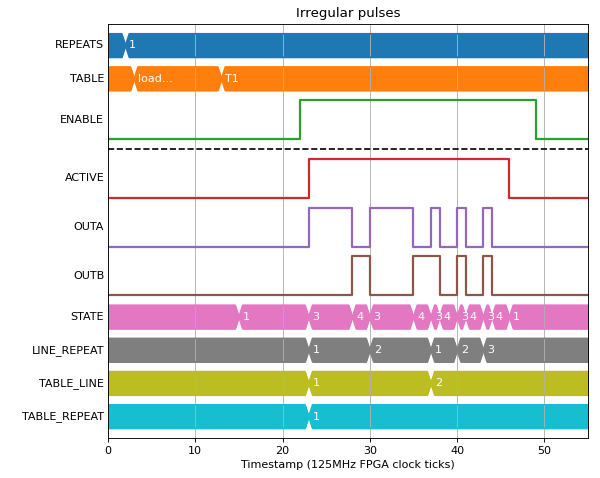
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
2 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
2 |
0 |
1 |
0 |
0 |
0 |
0 |
3 |
Immediate |
0 |
1 |
1 |
1 |
0 |
0 |
0 |
0 |
2 |
0 |
0 |
0 |
0 |
0 |
0 |
And we can set repeats on the entire table too. Note that in the second line of this table we have suppressed phase1 by setting its time to 0:
(Source code
, png
, hires.png
, pdf
)
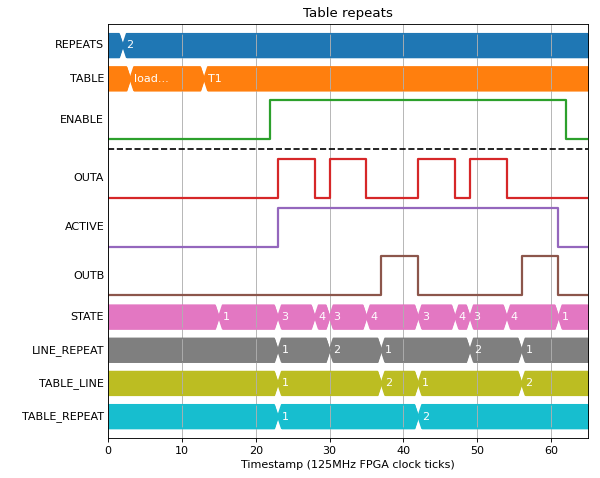
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
2 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
2 |
0 |
0 |
0 |
0 |
0 |
0 |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
5 |
0 |
1 |
0 |
0 |
0 |
0 |
There are 6 outputs which allow for complex patterns to be generated:
(Source code
, png
, hires.png
, pdf
)
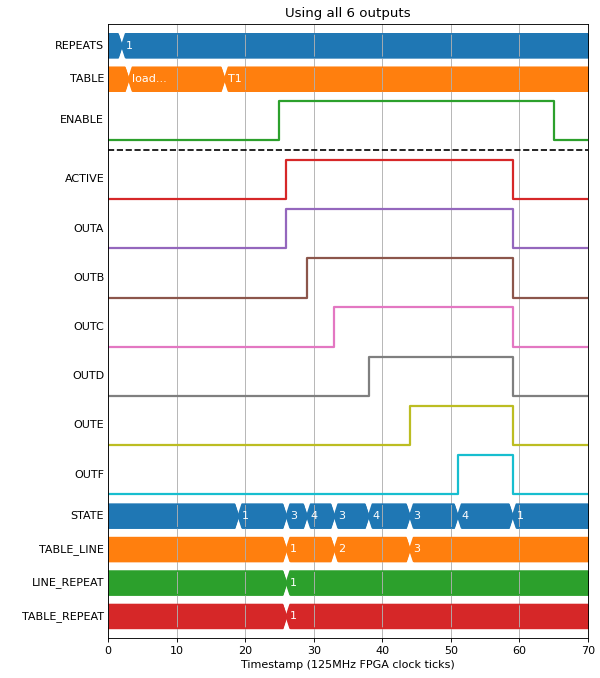
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
3 |
1 |
0 |
0 |
0 |
0 |
0 |
4 |
1 |
1 |
0 |
0 |
0 |
0 |
1 |
Immediate |
0 |
5 |
1 |
1 |
1 |
0 |
0 |
0 |
6 |
1 |
1 |
1 |
1 |
0 |
0 |
1 |
Immediate |
0 |
7 |
1 |
1 |
1 |
1 |
1 |
0 |
8 |
1 |
1 |
1 |
1 |
1 |
1 |
Statemachine
There is an internal statemachine that controls which phase is currently being output. It has a number of transitions that allow it to skip PHASE1 if there is none, or skip WAIT_TRIGGER if there is no trigger condition.
External trigger sources
The trigger column in the table allows an optional trigger condition to be waited on before the phased times are started. The trigger condition is checked on each repeat of the line, but not checked during phase1 and phase2. You can see when the Block is waiting for a trigger signal as it will enter the WAIT_TRIGGER(2) state:
(Source code
, png
, hires.png
, pdf
)
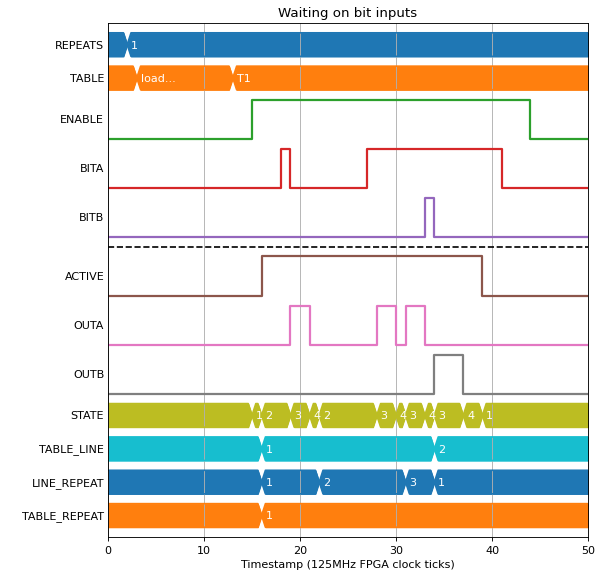
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
3 |
BITA=1 |
0 |
2 |
1 |
0 |
0 |
0 |
0 |
0 |
1 |
0 |
0 |
0 |
0 |
0 |
0 |
1 |
BITB=1 |
0 |
3 |
0 |
1 |
0 |
0 |
0 |
0 |
2 |
0 |
0 |
0 |
0 |
0 |
0 |
You can also use a position field as a trigger condition in the same way, this is useful to do a table based position compare:
(Source code
, png
, hires.png
, pdf
)
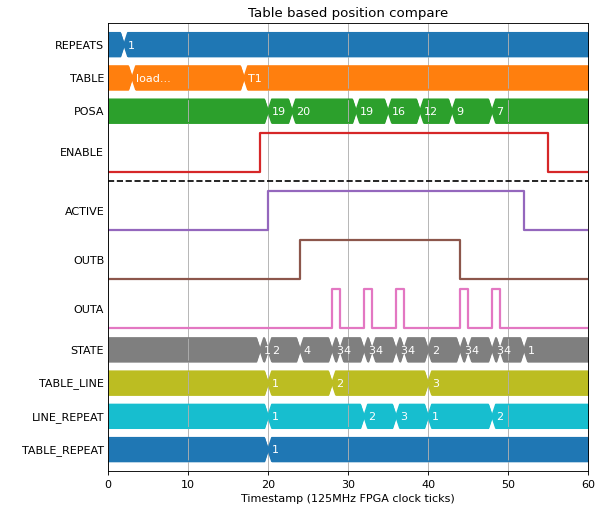
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
POSA>=POSITION |
20 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
4 |
0 |
1 |
0 |
0 |
0 |
0 |
3 |
Immediate |
0 |
1 |
1 |
1 |
0 |
0 |
0 |
0 |
3 |
0 |
1 |
0 |
0 |
0 |
0 |
2 |
POSA<=POSITION |
10 |
1 |
1 |
0 |
0 |
0 |
0 |
0 |
3 |
0 |
0 |
0 |
0 |
0 |
0 |
Prescaler
Each row of the table gives a time value for the phases. This value can be scaled with a block wide prescaler to allow a frame to be longer than 2**32 * 8e-9 = about 34 seconds. For example:
(Source code
, png
, hires.png
, pdf
)
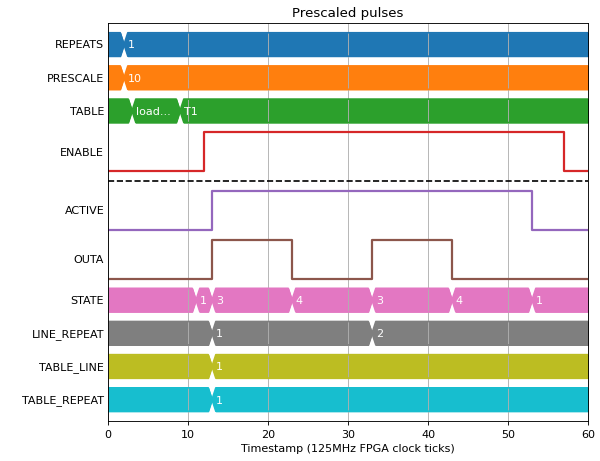
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
2 |
Immediate |
0 |
1 |
1 |
0 |
0 |
0 |
0 |
0 |
1 |
0 |
0 |
0 |
0 |
0 |
0 |
Interrupting a sequence
Setting the repeats on a table row to 0 will cause it to iterate until interrupted by a falling ENABLE signal:
(Source code
, png
, hires.png
, pdf
)
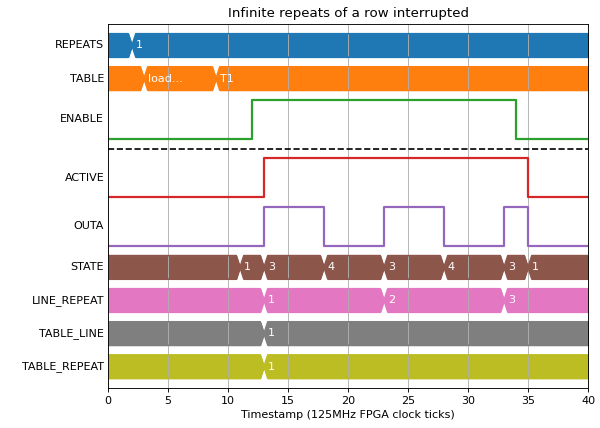
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
0 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
5 |
0 |
0 |
0 |
0 |
0 |
0 |
In a similar way, REPEATS=0 on a table will cause the whole table to be iterated until interrupted by a falling ENABLE signal:
(Source code
, png
, hires.png
, pdf
)
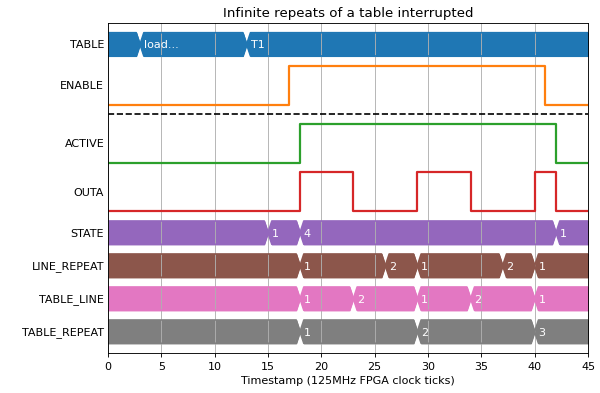
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
2 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
3 |
0 |
0 |
0 |
0 |
0 |
0 |
And a rising edge of the ENABLE will re-run the same table from the start:
(Source code
, png
, hires.png
, pdf
)
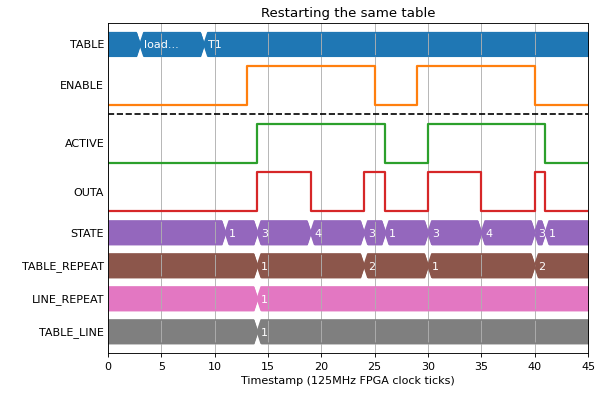
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
5 |
0 |
0 |
0 |
0 |
0 |
0 |
Table rewriting
If a table is written while enabled, the outputs and table state are reset and operation begins again from the first repeat of the first line of the table:
(Source code
, png
, hires.png
, pdf
)
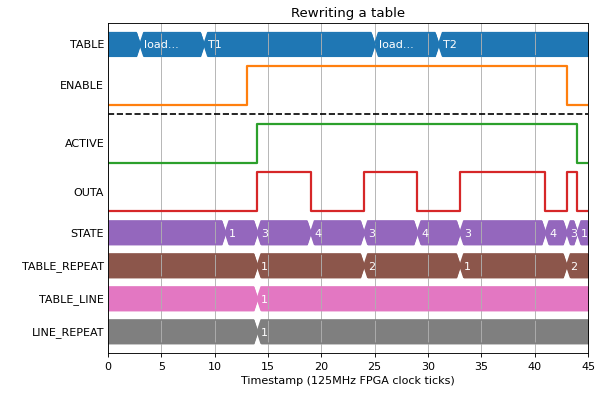
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
5 |
1 |
0 |
0 |
0 |
0 |
0 |
5 |
0 |
0 |
0 |
0 |
0 |
0 |
T2 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
8 |
1 |
0 |
0 |
0 |
0 |
0 |
2 |
0 |
0 |
0 |
0 |
0 |
0 |
Double table mode
A table ending with a zeroed entry indicates that there will be a next table, a new table can be pushed as soon as CAN_WRITE_NEXT becomes 1. The user is responsible to push a table that gives enough time to be able to push the next table before the last line is reached. The last table will not contain the last zeroed entry and will be the only table that will be repeated according to the REPEATS register.
(Source code
, png
, hires.png
, pdf
)
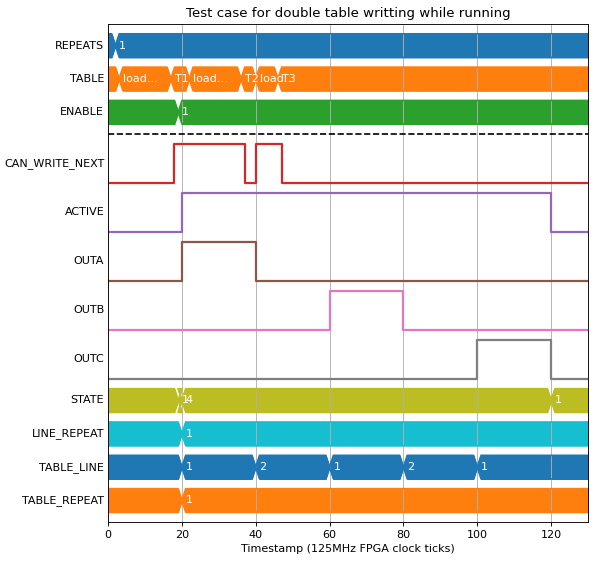
T1 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
20 |
1 |
0 |
0 |
0 |
0 |
0 |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
20 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
T2 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
20 |
0 |
1 |
0 |
0 |
0 |
0 |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
20 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
T3 |
||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
# |
Trigger |
Phase1 |
Phase1 Outputs |
Phase2 |
Phase2 Outputs |
|||||||||||
Repeats |
Condition |
Position |
Time |
A |
B |
C |
D |
E |
F |
Time |
A |
B |
C |
D |
E |
F |
1 |
Immediate |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
0 |
20 |
0 |
0 |
1 |
0 |
0 |
0 |